Grasping Python Classes: A Beginner's Journey to Inheritance
- Al Ahmad
- Oct 24, 2023
- 3 min read
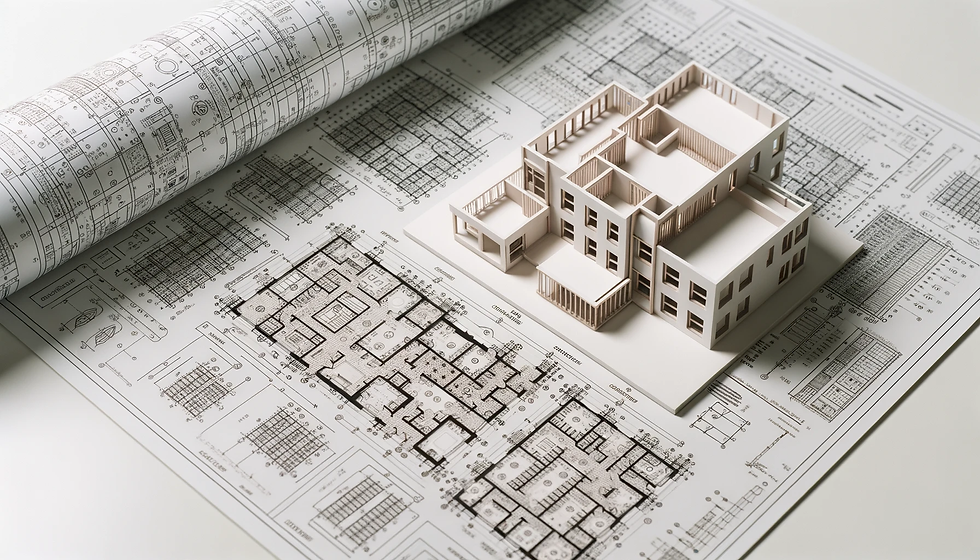
Introduction
Hey there, Python newbies! Ever wondered how Python makes everything so object-oriented? Well, you're about to find out! Especially if you've dabbled in Java before, Python's approach to Object-Oriented Programming (OOP) is like a breath of fresh air. We'll explore Python classes, objects, methods, and yes, the pièce de résistance—inheritance. So, fasten your seatbelts; we're going on a Pythonic adventure!
The ABCs of Python Classes
In Python, a class serves as a blueprint for creating objects, which are essentially instances of the class. Think of a class as a recipe and objects as the dishes made from that recipe. When you define a class in Python, you use the class keyword, followed by a name for the class, and a colon to indicate the beginning of the class body. Inside the class, you can define methods and attributes. One special method is __init__, which initializes the object's attributes when it's created. Here's how it works:
pythonCopy code
class Dog:
def __init__(self, name):
self.name = name # 'name' is an attribute of the Dog class
In this example, __init__ is a constructor method that gets called when you create a new Dog object. The self keyword refers to the object itself, and name is an attribute we're setting for each Dog object.
The Role of Methods in Python Classes
Methods are the functions that reside within a class and define the behaviors that an object can perform. In simpler terms, if a class is a blueprint, methods are the utilities like electricity and plumbing. For our Dog class, we can add a method called bark to make our dog objects more interactive:
pythonCopy code
def bark(self):
print(f"{self.name} goes Woof!")
Here, bark is a method that prints a message when called. To use this method, you first create an object of the Dog class and then call the bark method on that object.
The Magic of Inheritance
Inheritance is a cornerstone of Object-Oriented Programming (OOP) that allows a class to inherit properties and behaviors from another class. Imagine you have a general Dog class but want to create a more specific Poodle class. You can make the Poodle class inherit all the attributes and methods from the Dog class and add some more unique to Poodle:
pythonCopy code
class Poodle(Dog):
def strut(self):
print(f"{self.name} is strutting around!")
In this example, Poodle is a child class that inherits from the parent Dog class. The strut method is unique to the Poodle class.
Conclusion
Well done! You've ventured into the captivating realm of Python classes. From understanding the basics of class definitions to adding methods and embracing the power of inheritance, you're well on your way to Python proficiency. The key to mastery is practice, so go ahead and start coding your own classes!
FAQs
What is the __init__ method and why is it special? The __init__ method acts as a constructor in Python. It initializes the attributes of a class when a new object is created.
What does the self keyword do in Python classes? The self keyword refers to the instance of the class itself. It's used within methods to access attributes and other methods of the class.
How do methods differ from attributes in a class? Attributes are the characteristics or properties that an object has, while methods are the actions or behaviors the object can perform.
How do I call a method on an object? After creating an object from a class, you can call its methods using dot notation, like object.method().
What is inheritance and how does it work in Python? Inheritance allows a child class to inherit attributes and methods from a parent class. It's done using the class Child(Parent) syntax in Python.
Can a child class have its own unique methods? Yes, a child class can have methods that are unique to it, in addition to the methods it inherits from the parent class.
What's the difference between a parent class and a child class? A parent class is a general blueprint that can be inherited by child classes. A child class inherits attributes and methods from the parent and can also have its own unique features.
How important is practice in mastering Python classes? Practice is crucial. The more you code, the more comfortable you'll become with Python classes and OOP concepts.
Comentarios