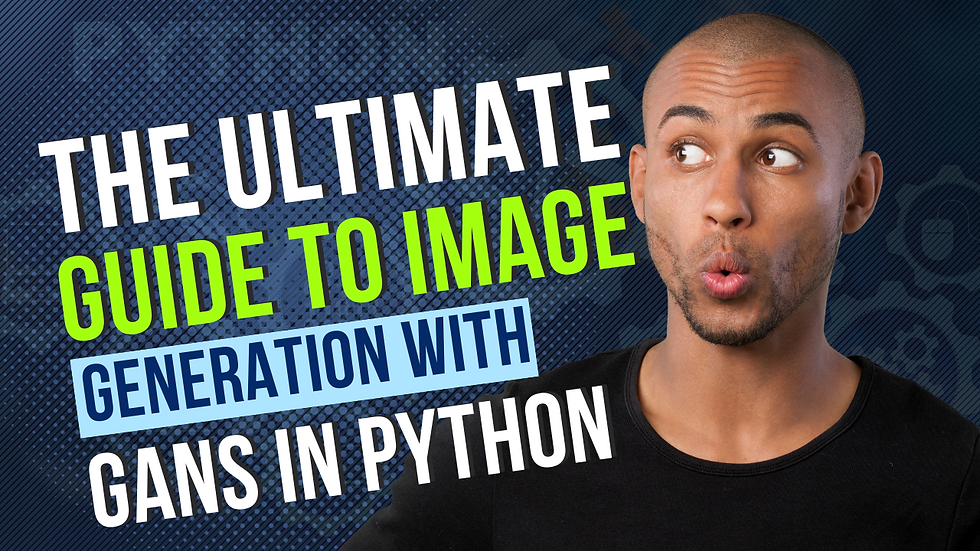
Introduction
Welcome to the definitive guide on Generative Adversarial Networks (GANs) for image generation in Python. Whether you're a beginner in machine learning or an experienced data scientist, this blog post is designed to provide you with the knowledge and tools to master image generation with GANs.
The Magic of GANs
Generative Adversarial Networks (GANs) have been nothing short of revolutionary in the field of machine learning.
GANs vs Traditional Methods
While traditional methods like neural style transfer and texture synthesis have their merits, GANs offer a level of realism and customization that is unparalleled.
The Inner Workings of GANs
The Generator aims to produce data that is indistinguishable from real data, while the Discriminator tries to differentiate between the two. This creates a fascinating dynamic where both networks improve over time, leading to increasingly convincing generated data.
Your First GAN Model
Before diving into advanced topics, let's start with a simple GAN model using PyTorch. This will give you a hands-on introduction to the world of GANs.
# Simple GAN Code Example
import torch
import torch.nn as nn
class SimpleGAN(nn.Module):
def __init__(self):
super(SimpleGAN, self).__init__()
self.fc1 = nn.Linear(100, 128)
self.fc2 = nn.Linear(128, 3)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = torch.sigmoid(self.fc2(x))
return x
generator = SimpleGAN()
noise = torch.randn(1, 100)
generated_img = generator(noise).detach().numpy().reshape(1, 3, 1)
Taking it to the Next Level: DCGANs
Deep Convolutional GANs (DCGANs) are an advanced form of GANs that use convolutional layers for better performance and quality. Below is a Python code example that demonstrates how to generate realistic grayscale images using DCGANs.
# Advanced DCGAN Code
import torch
import torch.nn as nn
import torch.optim as optim
import torchvision
import torchvision.transforms as transforms
# Hyperparameters
batch_size = 128
learning_rate = 0.0002
num_epochs = 100
latent_dim = 100
# Data loading
transform = transforms.Compose([transforms.ToTensor(), transforms.Normalize([0.5], [0.5])])
trainset = torchvision.datasets.MNIST(root='./data', train=True, download=True, transform=transform)
trainloader = torch.utils.data.DataLoader(trainset, batch_size=batch_size, shuffle=True)
# Generator
class Generator(nn.Module):
def __init__(self):
super(Generator, self).__init__()
self.main = nn.Sequential(
nn.ConvTranspose2d(latent_dim, 256, 4, 1, 0, bias=False),
nn.BatchNorm2d(256),
nn.ReLU(True),
nn.ConvTranspose2d(256, 128, 4, 2, 1, bias=False),
nn.BatchNorm2d(128),
nn.ReLU(True),
nn.ConvTranspose2d(128, 64, 4, 2, 1, bias=False),
nn.BatchNorm2d(64),
nn.ReLU(True),
nn.ConvTranspose2d(64, 1, 4, 2, 1, bias=False),
nn.Tanh()
)
def forward(self, x):
return self.main(x)
Real-world Applications of GANs
From creating realistic video game environments to generating medical imaging data, the applications of GANs are vast and varied.
FAQs
1. What are GANs?
- Generative Adversarial Networks (GANs) are a type of machine learning model that consists of two neural networks: the Generator and the Discriminator.
2. How do GANs work?
- The Generator creates fake data, while the Discriminator evaluates them. The two networks are trained together in an adversarial manner to improve their performance.
3. Are GANs better than traditional methods?
- GANs generally produce more realistic and customizable results compared to traditional methods like neural style transfer.
4. How do I start with GANs?
- You can start by understanding the basic principles and then move on to hands-on coding using frameworks like PyTorch or TensorFlow.
5. What are the applications of GANs?
- GANs have a wide range of applications including but not limited to art creation, medical imaging, and video game design.
6. What is the difference between a GAN and a DCGAN?
- DCGAN stands for Deep Convolutional GAN and it's an extension of the basic GAN with more layers and convolutional networks. It generally produces higher-quality results.
7. How do I choose the right hyperparameters for my GAN?
- Choosing the right hyperparameters is often a matter of trial and error, but common starting points include a learning rate of 0.0002 and a batch size of 128.
8. Can GANs be used for data augmentation?
- Yes, GANs can be used to augment datasets by generating additional data that is similar to the existing data.
9. What are the limitations of GANs?
- GANs require a lot of computational power and can sometimes be difficult to train. They can also sometimes produce artifacts in the generated images.
10. How can I optimize my GAN for better performance?
- Optimizing a GAN often involves fine-tuning the architecture, adjusting the learning rate, and sometimes using advanced techniques like gradient clipping.
11. What are some common problems when training GANs and how can they be solved?
- Common problems include mode collapse, vanishing gradients, and unstable training. Solutions often involve architectural tweaks and advanced training techniques.
Conclusion
GANs have opened up a new frontier in the world of machine learning and data science. With their ability to generate highly realistic data, they are transforming industries and creating endless possibilities for innovation.
Comments