Arrays Unleashed: A Comprehensive Guide to Using Arrays in Python
- Al Ahmad
- Feb 22, 2023
- 2 min read
Updated: Aug 18, 2023
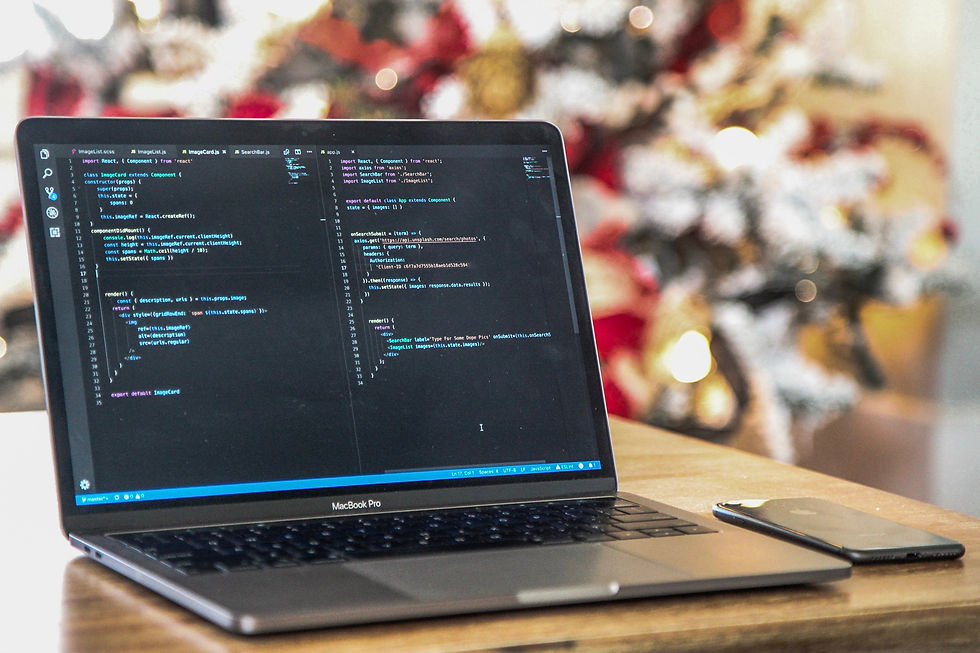
In this article, we will explore the topic of arrays in Python, a fundamental data structure used in programming. Arrays are used to store a collection of values, and they can be used to efficiently perform many operations. I will take you through the different types of arrays in Python and how to use them. By the end of this article, you will have a clear understanding of arrays in Python and their various applications. So, let's get started!
To begin with, let's talk about the most fundamental array in Python, the list. A list is a versatile and mutable array in Python that can hold elements of different data types. To create a list, simply use square brackets [] and separate the elements with commas. For example:
my_list = [1, 2, 3, "hello", True]
Here, we have a list that holds integers, strings, and a Boolean value. You can access elements of a list using their index, which starts at 0. For example:
print(my_list[0]) # Output: 1
print(my_list[3]) # Output: hello
Lists are incredibly flexible, and you can add or remove elements from them easily. To add an element to the end of a list, use the append() method. For example:
my_list.append(4)
print(my_list) # Output: [1, 2, 3, "hello", True, 4]
To remove an element from a list, use the remove() method. For example:
my_list.remove("hello")
print(my_list) # Output: [1, 2, 3, True, 4]
Another essential array in Python is the NumPy array. NumPy is a powerful library for scientific computing in Python, and its arrays are incredibly efficient for numerical operations. To use NumPy arrays, you need to install the NumPy library first. Here's how you can create a NumPy array:
import numpy as np
my_array = np.array([1, 2, 3, 4, 5])
NumPy arrays are much faster than lists when performing numerical operations. For example, let's say we want to calculate the square of each element in a list and a NumPy array. Here's how we can do it:
import time
my_list = [1, 2, 3, 4, 5]
my_array = np.array([1, 2, 3, 4, 5])
start_time = time.time()
squared_list = [num ** 2 for num in my_list]
print("Time taken for list:", time.time() - start_time)
start_time = time.time()
squared_array = my_array ** 2
print("Time taken for NumPy array:", time.time() - start_time)
The output of this code will be the time taken for each operation. You will notice that the NumPy array operation is much faster than the list operation.
There are many other arrays in Python, such as the array.array, which is a more efficient array for storing homogeneous data types, and the deque, which is a double-ended queue. You can also create your own custom arrays in Python using the array module.
In conclusion, arrays are a critical data structure in Python, and understanding their many types is essential for any programmer. Whether you're working with lists, NumPy arrays, or other types of arrays, make sure you use them to your advantage to optimize your code and make it more efficient.
Comentários