Mastering RPG Game Creation in Python: A Step-by-Step Guide for Beginners
- Al Ahmad
- Mar 17, 2023
- 5 min read
Updated: Aug 18, 2023
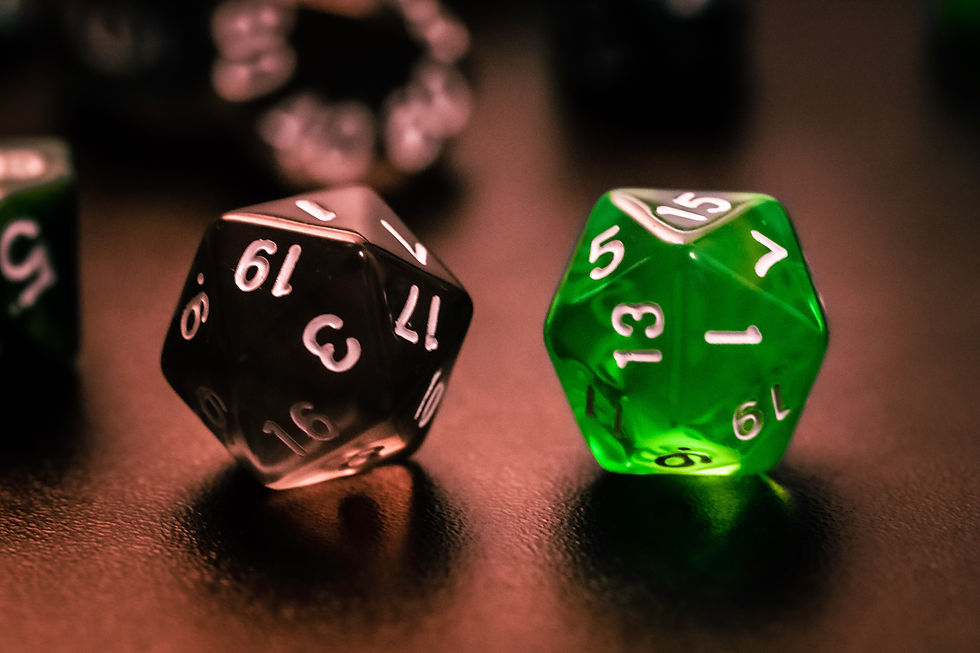
Introduction: Role-playing games, or RPGs, are popular types of games where players assume the roles of characters and explore a fictional world while completing quests and battling enemies. With the rise of game development tools and programming languages, it's now easier than ever to create your own RPG game. In this article, we'll guide you through the basic steps of creating an RPG game in Python, using examples and code snippets along the way.
Step 1: Set Up Your Development Environment Before you start creating your RPG game, you need to set up your development environment. This involves installing Python and any necessary libraries and tools, such as Pygame, a popular library for game development in Python. Here's an example of how to install Pygame using pip, a package installer for Python:
!pip install pygame
Step 2: Create the Game Window Once you've set up your development environment, you can start creating your game. The first step is to create the game window using Pygame. The game window is the visual interface that players will interact with. Here's an example of how to create a game window in Pygame:
import pygame
pygame.init()
width = 800
height = 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("My RPG Game")
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((255, 255, 255))
pygame.display.flip()
pygame.quit()
In this code snippet, we import the Pygame library and initialize it. We then set the width and height of the game window and create the game window using the pygame.display.set_mode() function. We also set the caption of the game window using the pygame.display.set_caption() function. Next, we create a loop that will keep the game window open until the player closes it. We use the pygame.event.get() function to get any events that occur in the game window, such as the player clicking the close button. If the player clicks the close button, we set the running variable to False, which will exit the game window loop. Inside the loop, we fill the game window with a white color using the screen.fill() function and update the game window using the pygame.display.flip() function. Finally, we quit Pygame using the pygame.quit() function. Step 3: Add Characters and Objects Now that we have the game window set up, we can start adding characters and objects to the game world. In an RPG game, characters are often represented as sprites, which are graphical images that move around the game world. Here's an example of how to add a character sprite to the game world using Pygame:
import pygame
pygame.init()
width = 800
height = 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("My RPG Game")
player_image = pygame.image.load("player.png")
player_rect = player_image.get_rect()
player_rect.center = (width // 2, height // 2)
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((255, 255, 255))
screen.blit(player_image, player_rect)
pygame.display.flip()
pygame.quit()
In this code snippet, we load a player sprite image using the pygame.image.load() function and get the sprite's rectangular dimensions using theget_rect()method. We then center the sprite on the game window using thecenter attribute. Inside the game window loop, we draw the sprite onto the game window using the screen.blit() function. This function takes the sprite image and its rectangular dimensions as parameters and draws it onto the game window. We also update the game window using the pygame.display.flip() function.
Step 4: Implement Game Mechanics Now that we have characters and objects in the game world, we can start implementing game mechanics. This involves creating game logic that defines how characters and objects interact with each other. Here's an example of how to implement player movement using the arrow keys in Pygame:
import pygame
pygame.init()
width = 800
height = 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("My RPG Game")
player_image = pygame.image.load("player.png")
player_rect = player_image.get_rect()
player_rect.center = (width // 2, height // 2)
player_speed = 5
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
player_rect.move_ip(-player_speed, 0)
if keys[pygame.K_RIGHT]:
player_rect.move_ip(player_speed, 0)
if keys[pygame.K_UP]:
player_rect.move_ip(0, -player_speed)
if keys[pygame.K_DOWN]:
player_rect.move_ip(0, player_speed)
screen.fill((255, 255, 255))
screen.blit(player_image, player_rect)
pygame.display.flip()
pygame.quit()
In this code snippet, we create a player_speed variable that defines how fast the player sprite can move. We then use the pygame.key.get_pressed() function to get a list of keys that are currently pressed. Inside the game window loop, we check if the left arrow key is pressed using the pygame.K_LEFT constant. If it is, we move the player sprite to the left using the move_ip() method. Similarly, we check if the right, up, and down arrow keys are pressed and move the player sprite accordingly. Step 5: Add Quests and Enemies Finally, we can add quests and enemies to the game world to make it more interesting and challenging for the player. Quests are objectives that the player must complete to progress in the game, while enemies are characters that the player must defeat to advance. Here's an example of how to add a quest and an enemy to the game world in Pygame:
import pygame
pygame.init()
width = 800
height = 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("My RPG Game")
player_image = pygame.image.load("player.png")
player_rect = player_image.get_rect()
player_rect.center = (width // 2, height // 2)
enemy_image = pygame.image.load("enemy.png")
enemy_rect = enemy_image.get_rect()
enemy_rect.center = (width // 2 + 50, height // 2)
quest_complete = False
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
player_rect.move_ip(-player_speed, 0)
if keys[pygame.K_RIGHT]:
player_rect.move_ip(player_speed, 0)
if keys[pygame.K_UP]:
player_rect.move_ip(0, -player_speed)
if keys[pygame.K_DOWN]:
player_rect.move_ip(0, player_speed)
if player_rect.colliderect(enemy_rect):
if not quest_complete:
print("You must complete the quest before fighting the enemy!")
else:
print("You defeated the enemy!")
running = False
if not quest_complete:
quest_rect = pygame.Rect(width // 2 - 25, height // 2 - 25, 50, 50)
pygame.draw.rect(screen, (255, 0, 0), quest_rect)
if player_rect.colliderect(quest_rect):
print("You completed the quest!")
quest_complete = True
screen.fill((255, 255, 255))
screen.blit(player_image, player_rect)
screen.blit(enemy_image, enemy_rect)
pygame.display.flip()
pygame.quit()
In this code snippet, we create an enemy_image variable and an enemy_rect variable to represent an enemy character. We set its initial position to the right of the player character. We also create a quest_complete variable that is initially set to False. Inside the game window loop, we check if the player collides with the enemy character using the colliderect() method. If the player has not completed the quest, we print a message telling the player to complete the quest first. If the player has completed the quest, we print a message telling the player that they have defeated the enemy and set the running variable to False to exit the game. We also add a quest objective to the game world using a pygame.Rect object and the pygame.draw.rect() function. If the player collides with the quest objective, we set the quest_complete variable to True and print a message telling the player that they have completed the quest. Conclusion Creating an RPG game in Python can be a challenging but rewarding experience. By following these basic steps, you can get started with creating your own RPG game using Pygame. Remember to start small and build up gradually, testing and debugging as you go along. With time and practice, you can create increasingly complex and engaging games that will keep players entertained for hours on end. Good luck!
Comments