The 35 Reserved keywords of Python: A Complete Guide with Examples
- Al Ahmad
- Feb 12, 2023
- 5 min read
Updated: Aug 18, 2023
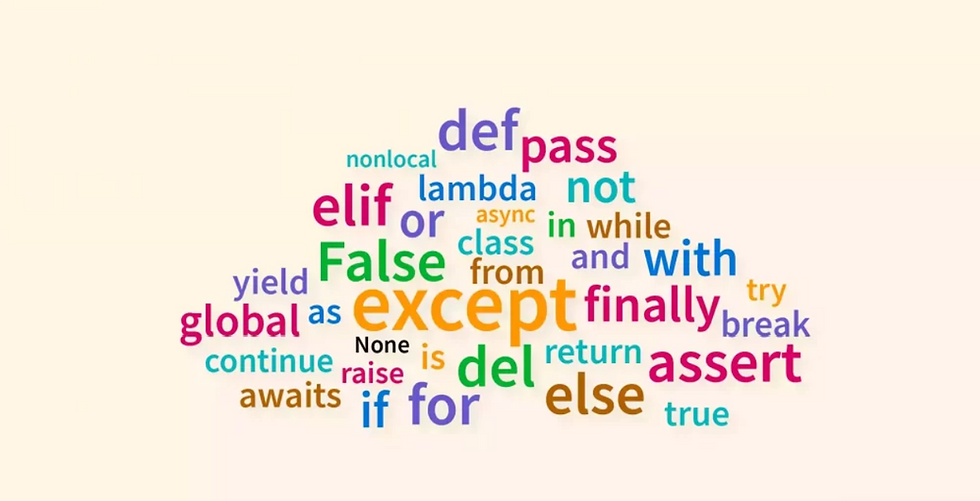
Python made its grand debut back in 1991 and has since skyrocketed to become one of the most beloved programming languages on the planet! Its creator, Guido van Rossum, had a vision to create a language that was simple, easy to read, and accessible to both beginners and seasoned coders alike.
The reserved keywords in Python play a crucial role in defining the language's syntax and structure. They're the building blocks for controlling the flow of your program, creating functions and classes, and manipulating data.
As Python evolved and expanded its capabilities, so too did the number of reserved keywords. For instance, Python 3.7 introduced the async and await keywords to support asynchronous programming, while the raise keyword has been a staple of the language since its earliest versions.
Each of Python's reserved keywords has a unique purpose, making them an indispensable part of the language. If you want to truly master Python, it's crucial to understand these keywords and how to use them.
Here is a list of the 35 reserved words in python with some basic examples for each.
and - Used to combine two boolean expressions, e.g. True and False returns False.
as - Used to create an alias for a module, e.g. import numpy as np.
assert - Used for debugging to check if a condition is true, e.g. assert x > 0.
async - Used to define an asynchronous function, e.g. async def fetch_data():.
await - Used inside an asynchronous function to wait for an asynchronous operation to complete, e.g. await fetch_data().
break - Used to exit a loop prematurely, e.g. for x in range(10): if x == 5: break.
class - Used to define a class, e.g. class Dog: pass.
continue - Used to skip the current iteration of a loop and move on to the next one, e.g. for x in range(10): if x % 2 == 0: continue.
def - Used to define a function, e.g. def add(a, b): return a + b.
del - Used to delete an object, e.g. del x.
elif - Used as a shorthand for else if, e.g. if x > 0: print("positive") elif x < 0: print("negative").
else - Used to specify a block of code to be executed if no conditions are met, e.g. if x > 0: print("positive") else: print("non-positive").
except - Used to catch and handle exceptions, e.g. try: 1/0 except ZeroDivisionError: print("division by zero").
False - A boolean value representing the absence of truth, e.g. if False: print("This won't print").
finally - Used to specify a block of code that will always be executed, regardless of exceptions, e.g. try: 1/0 finally: print("this will always print").
for - Used to iterate over a sequence (e.g. list, tuple, set, etc.), e.g. for x in [1, 2, 3]: print(x).
from - Used to import specific objects from a module, e.g. from math import sin, cos.
global - Used to declare a global variable, e.g. global x; x = 10.
if - Used to specify a block of code to be executed if a condition is true, e.g. if x > 0: print("positive").
import - Used to import a module, e.g. import math.
in - Used to check if an element is present in a sequence, e.g. if x in [1, 2, 3]: print("x is in the list").
is - Used to check if two variables are equal in both value and type, e.g. if type(x) is int: print("x is an integer").
lambda - Used to create small, anonymous functions in Python. These functions are known as "lambda functions" and are useful when you only need a simple function for a short period of time, e.g. sum = lambda x, y: x + y print(sum(3, 4))
None - Used to represent the default return value of a function that does not explicitly return any value. It can also be used as a placeholder value for variables that haven't been assigned a value yet, e.g. value = None print(value) # Output: None
nonlocal - Used in nested functions to indicate that a variable is not local to the inner function but instead refers to a variable in the nearest enclosing scope that is not global. e.g. def inner_function(): nonlocal x
not - is a logical operator in Python that returns the negation of a boolean expression. It can be used to invert the truth value of a boolean expression, e.g. x = True y = not x print(y)
or - is a logical operator in Python that returns True if either of the operands is True, e.g. x = False y = True z = x or y print(z)
pass - Used as a placeholder in Python that is used when a statement is required syntactically, but no action needs to be performed., e.g. def do_nothing(): pass
raise - Used to raise an exception in Python. It's typically used within a try-except block to signal that an error has occurred, e.g. def divide(a, b): if b == 0: raise ZeroDivisionError("division by zero") return a / b
return - Used to return a value from a function in Python, e.g. def square(x): return x * x
True - Used to represent boolean values, which are binary values that indicate true, e.g. value = True if value: print("Value is True") else: print("Value is False") # Output: Value is True
try - Used to catch exceptions in Python. It's typically used with the except keyword to specify what to do if an exception occurs, e.g. try: x = int("foo") except ValueError: print("Invalid input")
while - Used to create a loop in Python that repeats as long as a condition is True, e.g. i = 0 while i < 5: print(i) i += 1
with - Used to wrap the execution of a block of code with methods defined by a context manager, e.g. with open("file.txt", "r") as f: content = f.read() print(content)
yield - Used to create a generator in Python, e.g. def fibonacci(): a, b = 0, 1 while True: yield a a, b = b, a + b
Here's a simple Hangman game created with some of the keywords above
import random
def hangman(word):
wrong = 0
stages = ["",
"________ ",
"| ",
"| | ",
"| 0 ",
"| /|\ ",
"| / \ ",
"| "
]
rletters = list(word)
board = ["_"] * len(word)
win = False
print("Welcome to Hangman")
while wrong < len(stages) - 1:
print("\n")
msg = "Guess a letter: "
char = input(msg)
if char in rletters:
cind = rletters.index(char)
board[cind] = char
rletters[cind] = '$'
else:
wrong += 1
print((" ".join(board)))
e = wrong + 1
print("\n".join(stages[0: e]))
if "_" not in board:
print("You win!")
print(" ".join(board))
win = True
break
if not win:
print("\n".join(stages[0: wrong]))
print("You lose! It was {}.".format(word))
words = ["python", "java", "kotlin", "javascript", "ruby"]
word = random.choice(words)
hangman(word)
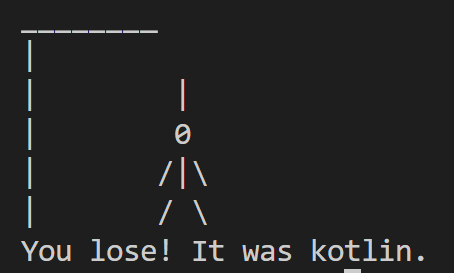
In conclusion, the reserved keywords in Python are crucial building blocks of the language. They play a critical role in defining the syntax and structure of the code and provide the means to control the flow of a program, create functions and classes, and perform operations on data. Understanding these keywords is vital for any programmer looking to master Python. With 35 reserved keywords at your disposal, there's no limit to the possibilities of what you can create. From basic programs to complex applications, these keywords offer a rich set of tools to accomplish your goals. Whether you're a beginner or an experienced programmer, taking the time to learn these keywords will pay off in the long run. In this article, we've explored each of the 35 reserved keywords in Python and provided simple examples to illustrate their use. By incorporating these keywords into your own code, you'll be well on your way to writing more efficient, readable, and effective programs. So, what are you waiting for? Get coding!
Comments