Unraveling the Mysteries of Tuples and Lists in Python
- Al Ahmad
- Mar 1, 2023
- 2 min read
Updated: Aug 18, 2023
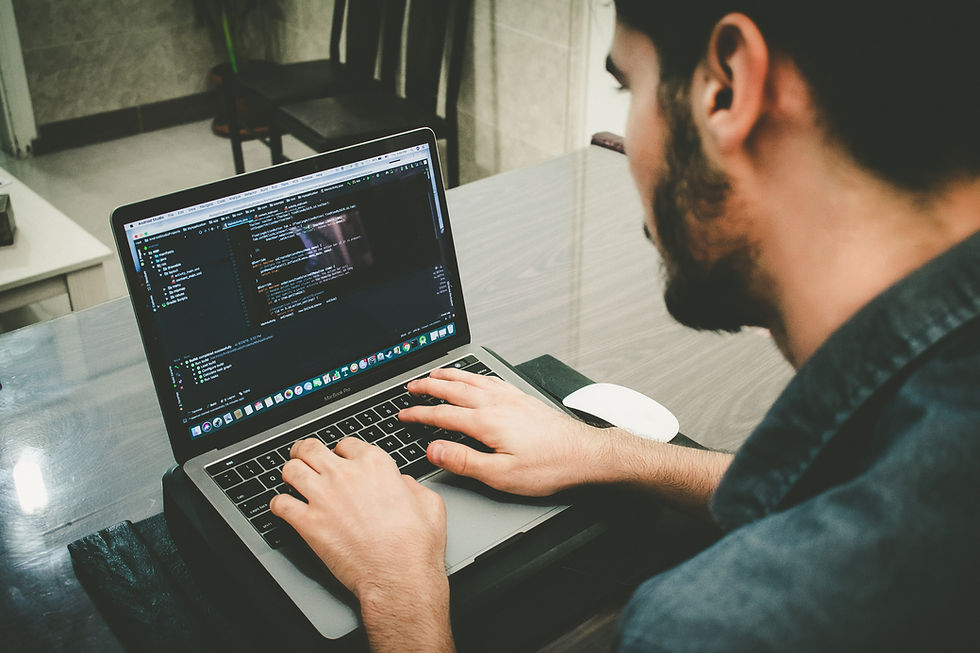
Tuples and lists are quintessential data structures in Python that can leave one baffled by their complexity and intricacies. If you're a neophyte programmer, it's natural to find these seemingly basic structures bewildering, as they can be both perplexing and fascinating simultaneously. In this article, we'll demystify these complex data structures and unravel their unique features, while adding a touch of flair and vigor.
Firstly, tuples and lists are both sequences of values in Python, but they differ fundamentally in their mutability. Tuples, being immutable, cannot be modified once created, whereas lists, being mutable, can be altered to add, remove or change their values. Moreover, tuples are renowned for their speed and memory efficiency, whereas lists offer a wider range of functionality.
Creating a tuple in Python involves using parentheses () and separating the values with commas. For instance:
pythonCopy code
my_tuple = (1, 2, 3, "hello", True)
Here, we created a tuple called my_tuple that encompasses five values: the integers 1, 2, and 3, the string "hello", and the boolean value True.
On the other hand, to create a list in Python, we use square brackets [] and separate the values with commas. For example:
pythonCopy code
my_list = [1, 2, 3, "hello", True]
In this example, we created a list called my_list that contains the same five values as the tuple above.
Now, let's delve into the remarkable features of tuples and lists in Python:
Accessing values: We can extract individual values from a tuple or list by referring to their index, which begins at 0. Here's an example:
pythonCopy code
print(my_tuple[0]) # Output: 1print(my_list[3]) # Output: hello
Slicing: We can also obtain a range of values in a tuple or list using slicing. For instance:
pythonCopy code
print(my_tuple[1:3]) # Output: (2, 3)print(my_list[:4]) # Output: [1, 2, 3, 'hello']
Adding values: We can incorporate values into a list using the append() method. Here's an example:
pythonCopy code
my_list.append("new value")
print(my_list) # Output: [1, 2, 3, 'hello', True, 'new value']
However, we cannot add values to a tuple once created.
Checking for values: We can validate if a value is in a tuple or list using in the keyword. Here's an example:
pythonCopy code
print(2 in my_tuple) # Output: Trueprint("world" in my_list) # Output: False
These are just a few examples of what you can do with tuples and lists in Python. As you continue to explore the intricacies of Python, you'll discover an array of fascinating ways to utilize these potent data structures in your programs.
In conclusion, tuples and lists are critical data structures in Python that possess unique features and characteristics.
Although they may appear daunting and complex, once you unravel their intricacies, they can be indispensable tools in writing efficient and effective Python code. By understanding the similarities and differences between tuples and lists, you can choose the appropriate data structure for your particular requirements and create programs that are both elegant and powerful.
Comments